Pydantic Schema生成指南:自定义JSON Schema
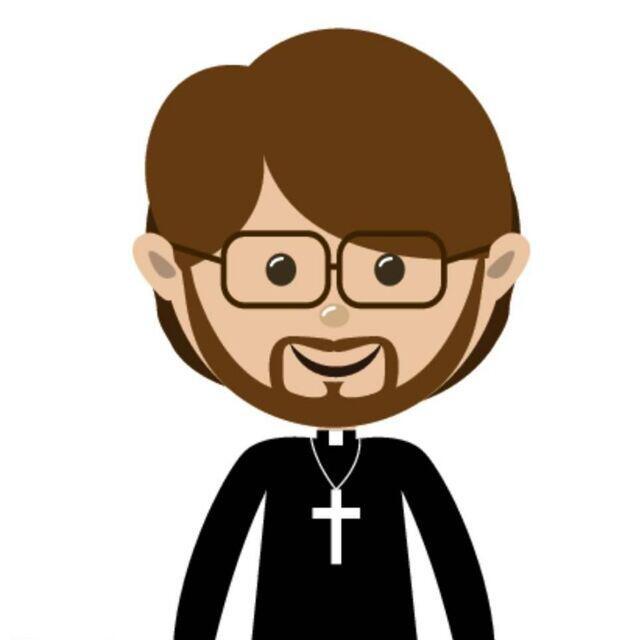

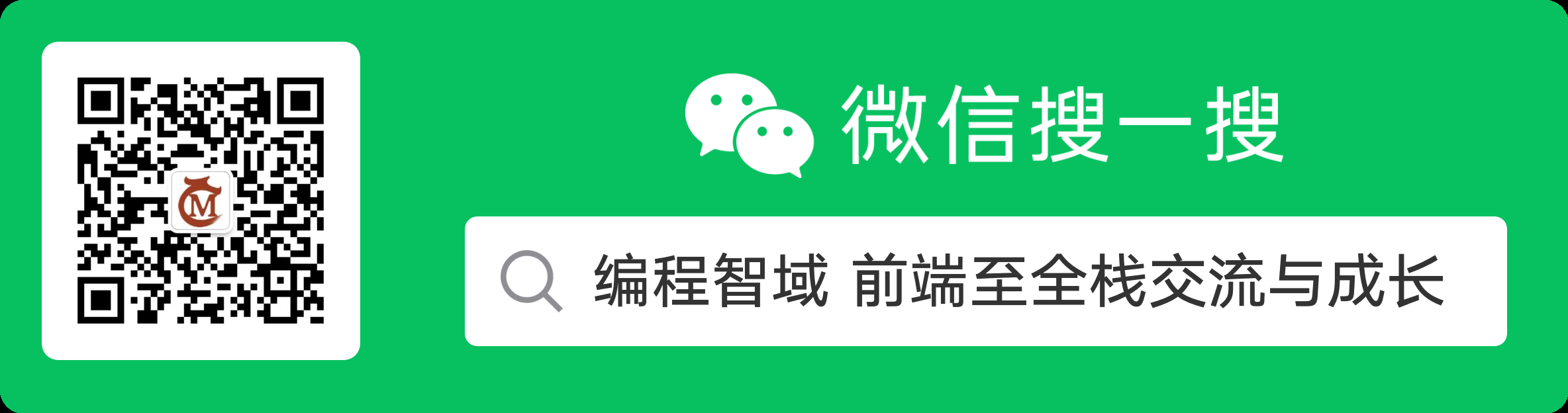
扫描二维码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
第一章:Schema生成基础
1.1 默认Schema生成机制
1 | from pydantic import BaseModel |
输出特征:
1 | { |
1.2 Schema生成流程
1 | graph TD |
第二章:核心定制技术
2.1 字段级元数据注入
1 | from pydantic import BaseModel, Field |
输出:
1 | { |
2.2 类型映射重载
1 | from pydantic import BaseModel |
第三章:动态Schema生成
3.1 运行时Schema构建
1 | from pydantic import create_model |
3.2 环境感知Schema
1 | from pydantic import BaseModel, ConfigDict |
第四章:企业级应用模式
4.1 OpenAPI增强方案
1 | from pydantic import BaseModel |
4.2 版本化Schema管理
1 | from pydantic import BaseModel, field_validator |
第五章:错误处理与优化
5.1 Schema验证错误
1 | try: |
5.2 性能优化策略
1 | from functools import lru_cache |
课后Quiz
Q1:如何添加自定义Schema属性?
A) 使用json_schema_extra
B) 修改全局配置
C) 继承GenerateJsonSchema
Q2:处理版本兼容的正确方式?
- 动态注入版本号
- 创建子类覆盖Schema
- 维护多个模型
Q3:优化Schema生成性能应使用?
- LRU缓存
- 增加校验步骤
- 禁用所有缓存
错误解决方案速查表
错误信息 | 原因分析 | 解决方案 |
---|---|---|
ValueError: 无效的format类型 | 不支持的Schema格式 | 检查字段类型与格式的兼容性 |
KeyError: 缺失必需字段 | 动态Schema未正确注入 | 验证__get_pydantic_json_schema__实现 |
SchemaGenerationError | 自定义生成器逻辑错误 | 检查类型映射逻辑 |
MemoryError | 大规模模型未缓存 | 启用模型Schema缓存 |
架构箴言:Schema设计应遵循”契约优先”原则,建议使用Git版本控制管理Schema变更,通过CI/CD流水线实现Schema的自动化测试与文档生成,建立Schema变更通知机制保障多团队协作。
余下文章内容请点击跳转至 个人博客页面 或者 扫码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
,阅读完整的文章:
往期文章归档:
- Pydantic递归模型深度校验36计:从无限嵌套到亿级数据的优化法则 | cmdragon’s Blog
- Pydantic异步校验器深:构建高并发验证系统 | cmdragon’s Blog
- Pydantic根校验器:构建跨字段验证系统 | cmdragon’s Blog
- Pydantic配置继承抽象基类模式 | cmdragon’s Blog
- Pydantic多态模型:用鉴别器构建类型安全的API接口 | cmdragon’s Blog
- FastAPI性能优化指南:参数解析与惰性加载 | cmdragon’s Blog
- FastAPI依赖注入:参数共享与逻辑复用 | cmdragon’s Blog
- FastAPI安全防护指南:构建坚不可摧的参数处理体系 | cmdragon’s Blog
- FastAPI复杂查询终极指南:告别if-else的现代化过滤架构 | cmdragon’s Blog
- FastAPI 核心机制:分页参数的实现与最佳实践 | cmdragon’s Blog
- FastAPI 错误处理与自定义错误消息完全指南:构建健壮的 API 应用 🛠️ | cmdragon’s Blog
- FastAPI 自定义参数验证器完全指南:从基础到高级实战 | cmdragon’s Blog
- FastAPI 参数别名与自动文档生成完全指南:从基础到高级实战 🚀 | cmdragon’s Blog
- FastAPI Cookie 和 Header 参数完全指南:从基础到高级实战 🚀 | cmdragon’s Blog
- FastAPI 表单参数与文件上传完全指南:从基础到高级实战 🚀 | cmdragon’s Blog
- FastAPI 请求体参数与 Pydantic 模型完全指南:从基础到嵌套模型实战 🚀 | cmdragon’s Blog
- FastAPI 查询参数完全指南:从基础到高级用法 🚀 | cmdragon’s Blog
- FastAPI 路径参数完全指南:从基础到高级校验实战 🚀 | cmdragon’s Blog
- FastAPI路由专家课:微服务架构下的路由艺术与工程实践 🌐 | cmdragon’s Blog
- FastAPI路由与请求处理进阶指南:解锁企业级API开发黑科技 🔥 | cmdragon’s Blog
- FastAPI路由与请求处理全解:手把手打造用户管理系统 🔌 | cmdragon’s Blog
- FastAPI极速入门:15分钟搭建你的首个智能API(附自动文档生成)🚀 | cmdragon’s Blog
- HTTP协议与RESTful API实战手册(终章):构建企业级API的九大秘籍 🔐 | cmdragon’s Blog
- HTTP协议与RESTful API实战手册(二):用披萨店故事说透API设计奥秘 🍕 | cmdragon’s Blog
- 从零构建你的第一个RESTful API:HTTP协议与API设计超图解指南 🌐 | cmdragon’s Blog
- Python异步编程进阶指南:破解高并发系统的七重封印 | cmdragon’s Blog
- Python异步编程终极指南:用协程与事件循环重构你的高并发系统 | cmdragon’s Blog
- Python类型提示完全指南:用类型安全重构你的代码,提升10倍开发效率 | cmdragon’s Blog
- 三大平台云数据库生态服务对决 | cmdragon’s Blog
- 分布式数据库解析 | cmdragon’s Blog
- 深入解析NoSQL数据库:从文档存储到图数据库的全场景实践 | cmdragon’s Blog
- 数据库审计与智能监控:从日志分析到异常检测 | cmdragon’s Blog