Pydantic模型继承解析:从字段继承到多态模型
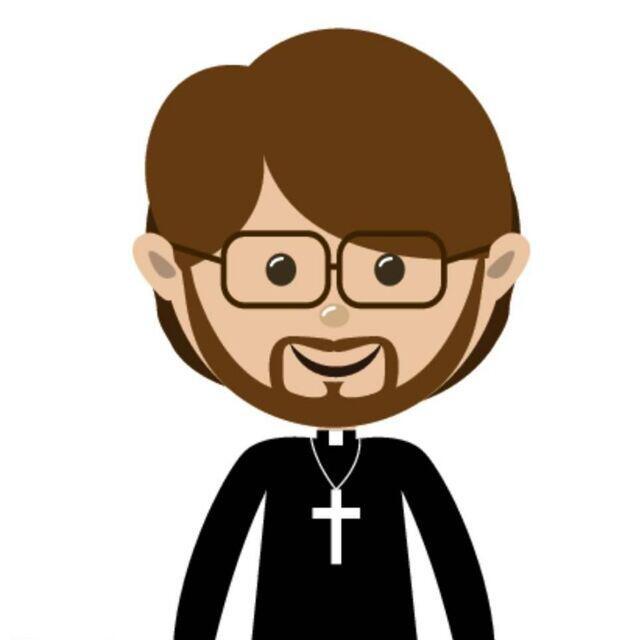
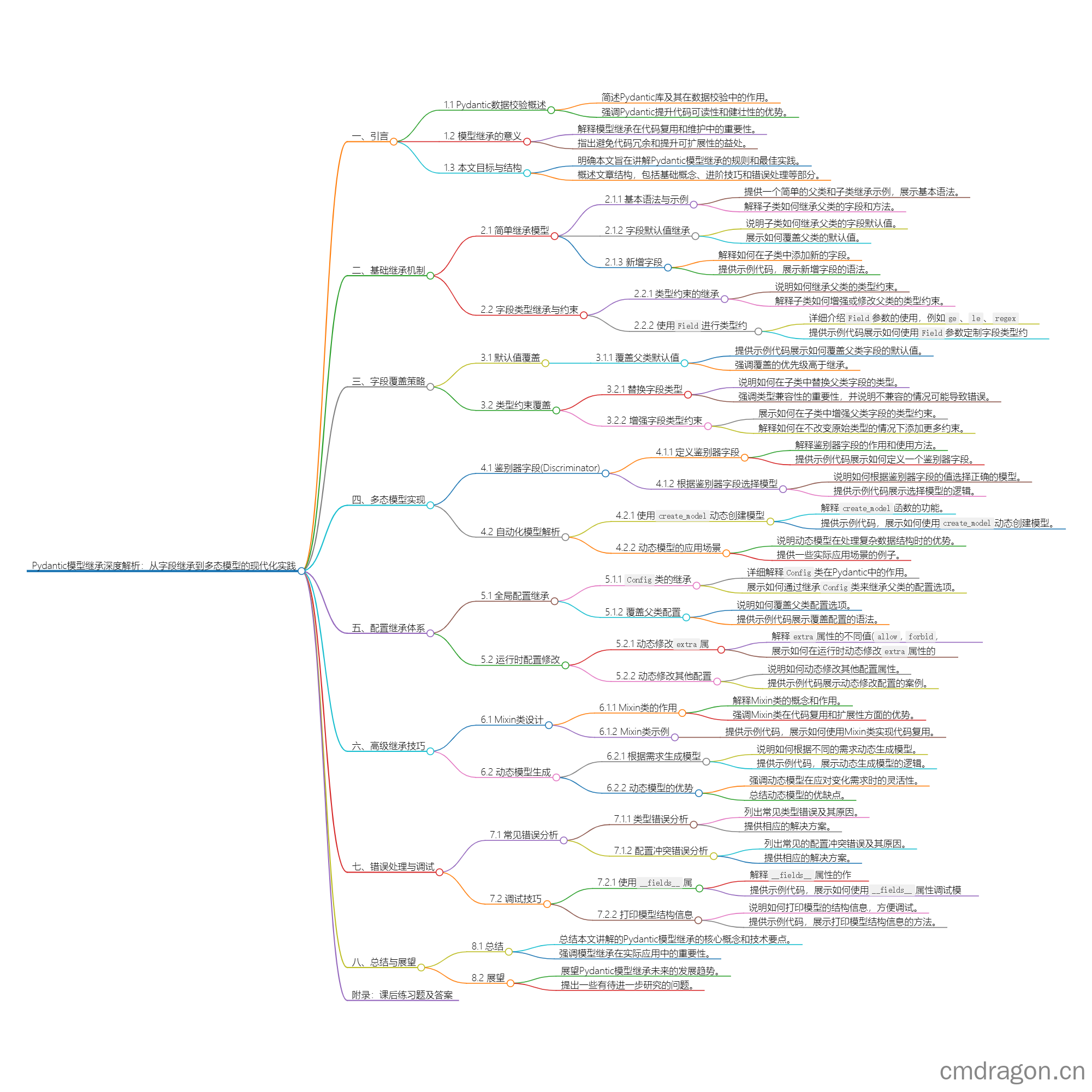
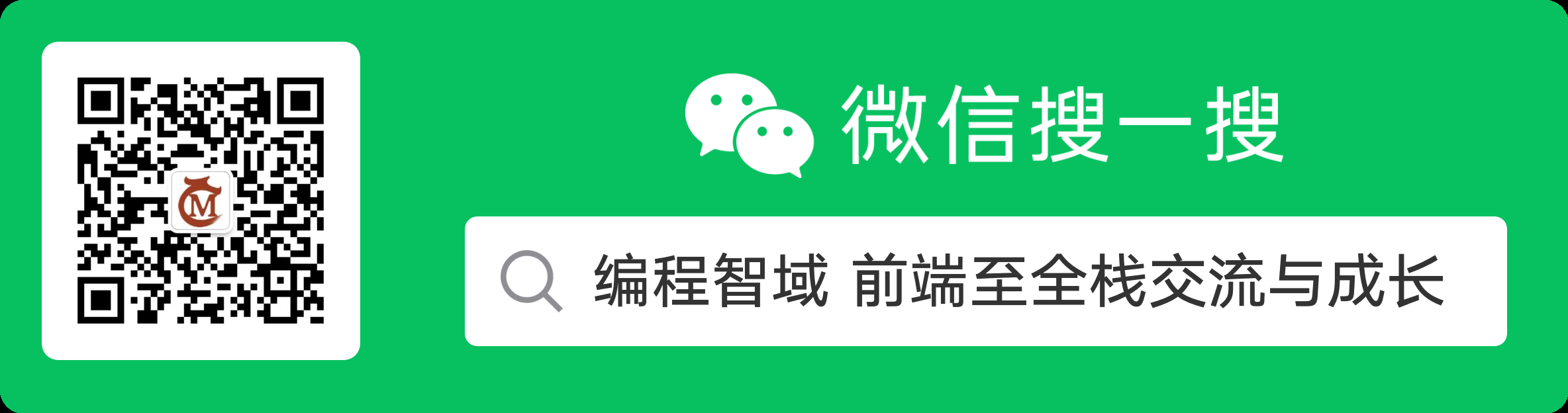
扫描二维码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
第一章:基础继承机制
1.1 简单继承模型
1 | from pydantic import BaseModel |
继承规则:
- 子类自动获得父类所有字段
- 字段默认值可被覆盖
- 新增字段需明确声明
1.2 字段类型强化
1 | from pydantic import Field |
第二章:字段覆盖策略
2.1 默认值覆盖
1 | class ConfigBase(BaseModel): |
2.2 类型约束升级
1 | class PaymentBase(BaseModel): |
覆盖规则矩阵:
父类字段定义 | 子类合法操作 | 非法操作 |
---|---|---|
str | 添加regex约束 | 更改为int类型 |
Optional[int] | 改为int | 改为str |
float | 添加ge/le约束 | 移除类型约束 |
第三章:多态模型实现
3.1 鉴别器字段
1 | from pydantic import Field |
3.2 自动化模型解析
1 | from pydantic import create_model |
第四章:配置继承体系
4.1 全局配置继承
1 | class Parent(BaseModel): |
配置继承规则:
- 使用
Config(Parent.Config)
显式继承 - 未指定时默认不继承父类配置
- 支持多级配置覆盖
4.2 运行时配置修改
1 | from pydantic import BaseModel, Extra |
第五章:高级继承技巧
5.1 Mixin类设计
1 | class TimestampMixin(BaseModel): |
5.2 动态模型生成
1 | def create_model_with_extra_fields(base: Type[BaseModel], **fields): |
第六章:错误处理与调试
6.1 继承错误分析
1 | try: |
常见错误码:
错误类型 | 触发场景 | 解决方案 |
---|---|---|
ValidationError | 字段类型不匹配 | 检查继承链中的类型定义 |
TypeError | 不兼容字段覆盖 | 使用@validator处理转型逻辑 |
ConfigConflict | 配置项冲突 | 显式指定配置继承关系 |
6.2 调试继承体系
1 | def print_model_fields(model: Type[BaseModel]): |
课后Quiz
Q1:如何实现字段默认值覆盖?
A) 在子类重新声明字段
B) 使用Field(default=…)
C) 修改父类定义
Q2:多态模型必须包含什么特征?
- 鉴别器字段
- 相同字段数量
- 统一校验规则
Q3:处理类型冲突的最佳方式?
- 使用@validator进行数据转换
- 强制类型转换
- 忽略类型检查
错误解决方案速查表
错误信息 | 原因分析 | 解决方案 |
---|---|---|
field type mismatch | 子类字段类型与父类不兼容 | 使用Union类型或添加转型校验器 |
extra fields not permitted | 未正确继承extra配置 | 显式继承父类Config |
discriminator field missing | 未定义多态鉴别器字段 | 添加带有别名_type的公共字段 |
扩展阅读
- 《Pydantic官方文档-模型继承》 - 官方标准实现规范
- 《类型系统设计模式》 - 企业级模型架构方案
- 《Python元编程实战》 - 动态模型生成技术
开发箴言:优秀的模型继承设计应遵循LSP(里氏替换原则),任何父类出现的地方都可以被子类替换。建议继承层级不超过3层,复杂场景优先选择组合模式而非深度继承。
余下文章内容请点击跳转至 个人博客页面 或者 扫码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
,阅读完整的文章:
往期文章归档:
- FastAPI性能优化指南:参数解析与惰性加载 | cmdragon’s Blog
- FastAPI依赖注入:参数共享与逻辑复用 | cmdragon’s Blog
- FastAPI安全防护指南:构建坚不可摧的参数处理体系 | cmdragon’s Blog
- FastAPI复杂查询终极指南:告别if-else的现代化过滤架构 | cmdragon’s Blog
- FastAPI 核心机制:分页参数的实现与最佳实践 | cmdragon’s Blog
- FastAPI 错误处理与自定义错误消息完全指南:构建健壮的 API 应用 🛠️ | cmdragon’s Blog
- FastAPI 自定义参数验证器完全指南:从基础到高级实战 | cmdragon’s Blog
- FastAPI 参数别名与自动文档生成完全指南:从基础到高级实战 🚀 | cmdragon’s Blog
- FastAPI Cookie 和 Header 参数完全指南:从基础到高级实战 🚀 | cmdragon’s Blog
- FastAPI 表单参数与文件上传完全指南:从基础到高级实战 🚀 | cmdragon’s Blog
- FastAPI 请求体参数与 Pydantic 模型完全指南:从基础到嵌套模型实战 🚀 | cmdragon’s Blog
- FastAPI 查询参数完全指南:从基础到高级用法 🚀 | cmdragon’s Blog
- FastAPI 路径参数完全指南:从基础到高级校验实战 🚀 | cmdragon’s Blog
- FastAPI路由专家课:微服务架构下的路由艺术与工程实践 🌐 | cmdragon’s Blog
- FastAPI路由与请求处理进阶指南:解锁企业级API开发黑科技 🔥 | cmdragon’s Blog
- FastAPI路由与请求处理全解:手把手打造用户管理系统 🔌 | cmdragon’s Blog
- FastAPI极速入门:15分钟搭建你的首个智能API(附自动文档生成)🚀 | cmdragon’s Blog
- HTTP协议与RESTful API实战手册(终章):构建企业级API的九大秘籍 🔐 | cmdragon’s Blog
- HTTP协议与RESTful API实战手册(二):用披萨店故事说透API设计奥秘 🍕 | cmdragon’s Blog
- 从零构建你的第一个RESTful API:HTTP协议与API设计超图解指南 🌐 | cmdragon’s Blog
- Python异步编程进阶指南:破解高并发系统的七重封印 | cmdragon’s Blog
- Python异步编程终极指南:用协程与事件循环重构你的高并发系统 | cmdragon’s Blog
- Python类型提示完全指南:用类型安全重构你的代码,提升10倍开发效率 | cmdragon’s Blog
- 三大平台云数据库生态服务对决 | cmdragon’s Blog
- 分布式数据库解析 | cmdragon’s Blog
- 深入解析NoSQL数据库:从文档存储到图数据库的全场景实践 | cmdragon’s Blog
- 数据库审计与智能监控:从日志分析到异常检测 | cmdragon’s Blog
- 数据库加密全解析:从传输到存储的安全实践 | cmdragon’s Blog
- 数据库安全实战:访问控制与行级权限管理 | cmdragon’s Blog
- 数据库扩展之道:分区、分片与大表优化实战 | cmdragon’s Blog
- 查询优化:提升数据库性能的实用技巧 | cmdragon’s Blog
- 性能优化与调优:全面解析数据库索引 | cmdragon’s Blog
- 存储过程与触发器:提高数据库性能与安全性的利器 | cmdragon’s Blog
- 数据操作与事务:确保数据一致性的关键 | cmdragon’s Blog