Tortoise-ORM级联查询与预加载性能优化
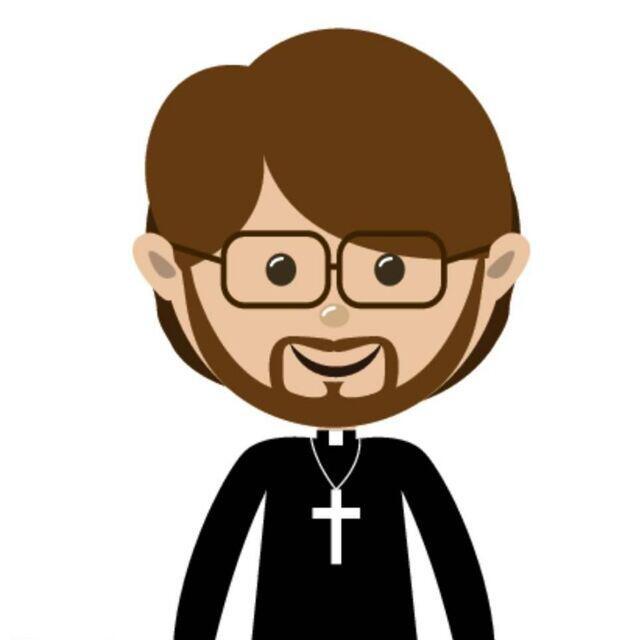
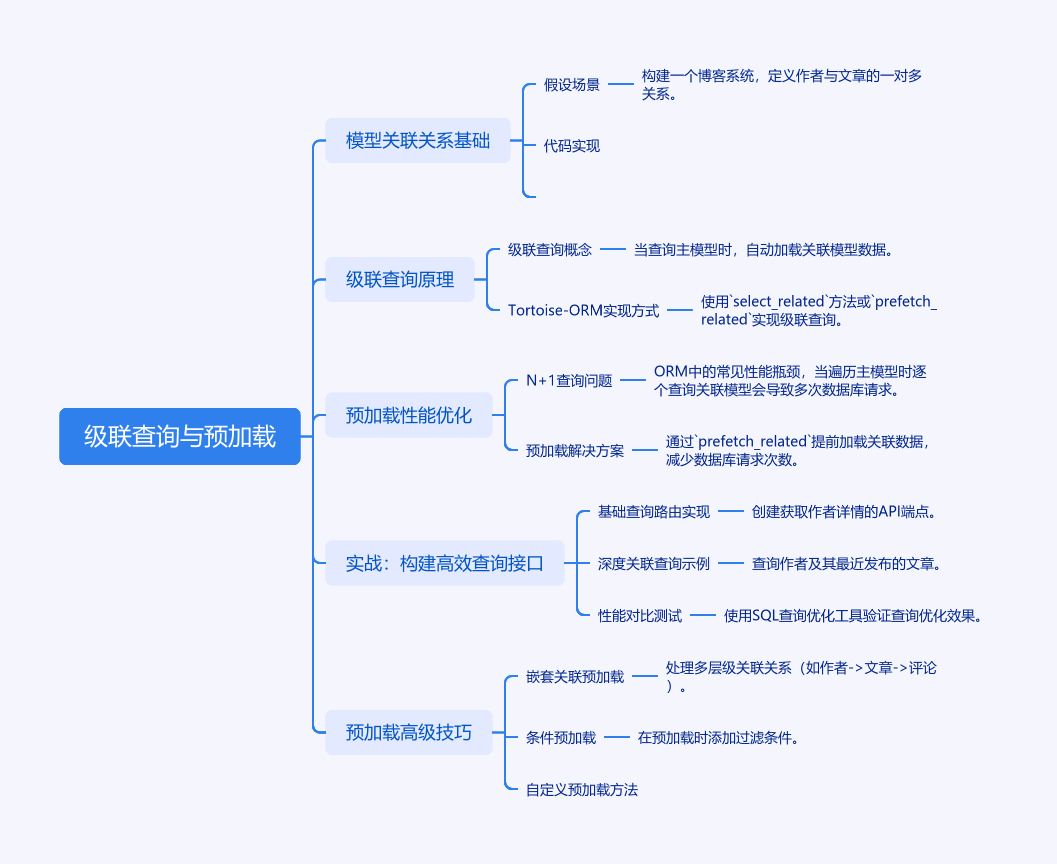
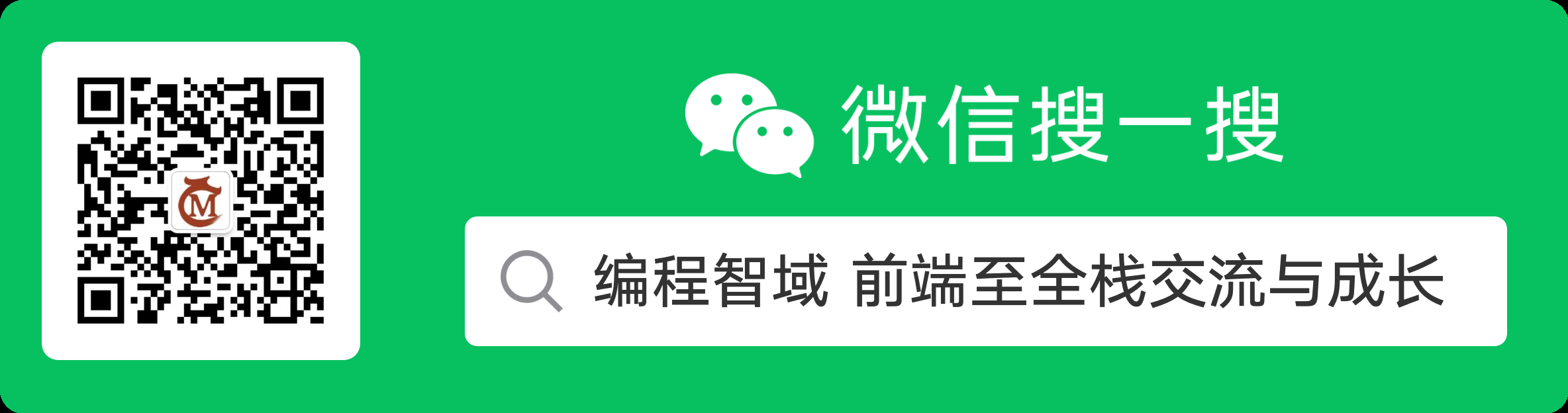
扫描二维码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
探索数千个预构建的 AI 应用,开启你的下一个伟大创意:https://tools.cmdragon.cn/
一、级联查询与预加载核心概念
在开发Web应用时,处理数据库表之间的关联关系是常见需求。Tortoise-ORM通过异步方式实现级联查询与预加载机制,能够显著提升API性能。
1.1 模型关联关系基础
假设我们构建一个博客系统,定义作者(Author)与文章(Article)的一对多关系:
1 | from tortoise.models import Model |
1.2 级联查询原理
当查询主模型时自动加载关联模型数据,例如获取作者时联带查询其所有文章。Tortoise-ORM通过select_related
方法实现:
1 | # 获取作者及其所有文章(单次查询) |
1.3 预加载性能优化
N+1查询问题是ORM常见性能瓶颈。当遍历作者列表时逐个查询文章会导致多次数据库请求。通过prefetch_related
提前加载关联数据:
1 | # 批量获取作者列表及其关联文章(2次查询) |
二、实战:构建高效查询接口
2.1 基础查询路由实现
创建获取作者详情的API端点:
1 | from fastapi import APIRouter |
2.2 深度关联查询示例
查询作者及其最近发布的3篇文章:
1 | class ArticlePreview(BaseModel): |
2.3 性能对比测试
使用EXPLAIN ANALYZE
验证查询优化效果:
1 | -- 未优化查询 |
三、预加载高级技巧
3.1 嵌套关联预加载
处理多层级关联关系(作者->文章->评论):
1 | # 三层级预加载示例 |
3.2 条件预加载
预加载时添加过滤条件:
1 | # 只预加载2023年发布的文章 |
3.3 自定义预加载方法
创建复杂查询的复用方法:
1 | class Author(Model): |
四、课后Quiz
当需要加载作者及其所有文章的标签时,正确的预加载方式是:
A)prefetch_related("articles")
B)prefetch_related("articles__tags")
C)select_related("articles.tags")
以下哪种场景最适合使用select_related?
A) 获取用户基本信息
B) 获取用户及其个人资料(一对一关系)
C) 获取博客及其所有评论(一对多关系)
答案与解析:
- B正确,双下划线语法用于跨模型预加载。C语法错误,select_related不能用于一对多关系
- B正确,select_related优化一对一关系查询。一对多用prefetch_related更合适
五、常见报错处理
报错1:RelationNotFoundError
原因:模型未正确定义关联字段
解决方案:
- 检查
related_name
拼写是否正确 - 确认关联模型已正确导入
报错2:QueryTimeoutError
原因:复杂预加载导致查询过慢
解决方案:
- 添加数据库索引
- 拆分查询为多个步骤
- 使用
only()
限制返回字段
报错3:ValidationError
原因:Pydantic模型字段不匹配
解决方案:
- 检查response_model字段类型
- 使用
orm_mode = True
配置 - 验证数据库字段类型是否匹配
六、最佳实践建议
- 始终在测试环境进行
EXPLAIN
查询分析 - 对频繁访问的接口添加Redis缓存层
- 为常用查询字段添加数据库索引
- 使用分页限制返回数据量
- 定期进行慢查询日志分析
安装环境要求:
1 | pip install fastapi uvicorn tortoise-orm pydantic |
配置Tortoise-ORM示例:
1 | from tortoise import Tortoise |
余下文章内容请点击跳转至 个人博客页面 或者 扫码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
,阅读完整的文章:
往期文章归档:
- 使用Tortoise-ORM和FastAPI构建评论系统 | cmdragon’s Blog
- 分层架构在博客评论功能中的应用与实现 | cmdragon’s Blog
- 深入解析事务基础与原子操作原理 | cmdragon’s Blog
- 掌握Tortoise-ORM高级异步查询技巧 | cmdragon’s Blog
- FastAPI与Tortoise-ORM实现关系型数据库关联 | cmdragon’s Blog
- Tortoise-ORM与FastAPI集成:异步模型定义与实践 | cmdragon’s Blog
- 异步编程与Tortoise-ORM框架 | cmdragon’s Blog
- FastAPI数据库集成与事务管理 | cmdragon’s Blog
- FastAPI与SQLAlchemy数据库集成 | cmdragon’s Blog
- FastAPI与SQLAlchemy数据库集成与CRUD操作 | cmdragon’s Blog
- FastAPI与SQLAlchemy同步数据库集成 | cmdragon’s Blog
- SQLAlchemy 核心概念与同步引擎配置详解 | cmdragon’s Blog
- FastAPI依赖注入性能优化策略 | cmdragon’s Blog
- FastAPI安全认证中的依赖组合 | cmdragon’s Blog
- FastAPI依赖注入系统及调试技巧 | cmdragon’s Blog
- FastAPI依赖覆盖与测试环境模拟 | cmdragon’s Blog
- FastAPI中的依赖注入与数据库事务管理 | cmdragon’s Blog
- FastAPI依赖注入实践:工厂模式与实例复用的优化策略 | cmdragon’s Blog
- FastAPI依赖注入:链式调用与多级参数传递 | cmdragon’s Blog
- FastAPI依赖注入:从基础概念到应用 | cmdragon’s Blog
- FastAPI中实现动态条件必填字段的实践 | cmdragon’s Blog
- FastAPI中Pydantic异步分布式唯一性校验 | cmdragon’s Blog
- 掌握FastAPI与Pydantic的跨字段验证技巧 | cmdragon’s Blog
- FastAPI中的Pydantic密码验证机制与实现 | cmdragon’s Blog
- 深入掌握FastAPI与OpenAPI规范的高级适配技巧 | cmdragon’s Blog
- Pydantic字段元数据指南:从基础到企业级文档增强 | cmdragon’s Blog
- Pydantic Schema生成指南:自定义JSON Schema | cmdragon’s Blog
- Pydantic递归模型深度校验36计:从无限嵌套到亿级数据的优化法则 | cmdragon’s Blog
- Pydantic异步校验器深:构建高并发验证系统 | cmdragon’s Blog
- Pydantic根校验器:构建跨字段验证系统 | cmdragon’s Blog
- Pydantic配置继承抽象基类模式 | cmdragon’s Blog
- Pydantic多态模型:用鉴别器构建类型安全的API接口 | cmdragon’s Blog
- FastAPI性能优化指南:参数解析与惰性加载 | cmdragon’s Blog
- FastAPI依赖注入:参数共享与逻辑复用 | cmdragon’s Blog